Dagger Android Tutorial 4
How to do dagger-android
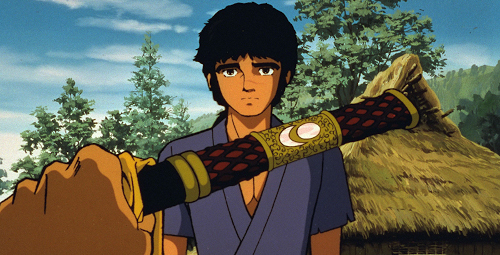
Convert RxJava stream into LiveData stream
When we use Rxjava for handling api requests, it will return a RxJava Stream. But in the presentation layer we are using LiveData, this article shows you how to make them work together.
The tool we are using here is LiveDataReactiveStreams.
It is an adapter from LiveData to ReactiveStream and vice versa.
This is how we do it in AuthViewModel
.
public class AuthViewModel extends ViewModel {
private static final String TAG = "AuthViewModel";
private final AuthApi authApi;
// 1. Instead of MutableLiveData, we declare a MediatorLiveData here.
private MediatorLiveData<User> authUser = new MediatorLiveData<>();
@Inject
AuthViewModel(AuthApi authApi) {
this.authApi = authApi;
}
void authenticateWith(int userId) {
//2. Convert the rx stream into livedata stream
final LiveData<User> source = LiveDataReactiveStreams.fromPublisher(authApi.getUser(userId).subscribeOn(Schedulers.io()));
//3. Add the converted stream as a source to the MediatorLiveData
authUser.addSource(source, new Observer<User>() {
@Override
public void onChanged(User user) {
authUser.setValue(user);
//4. Unsubscribe after we get the result
authUser.removeSource(source);
}
});
}
LiveData<User> user() {
return authUser;
}
}
Then in AuthActivity
:
Trigger login with user id.
private void attemptLogin() {
if (TextUtils.isEmpty(userIdEditText.getText().toString())) {
return;
}
viewModel.authenticateWith(Integer.parseInt(userIdEditText.getText().toString()));
}
Listening to the user live data.
private void subscribeObservers() {
viewModel.user().observe(this, new Observer<User>() {
@Override
public void onChanged(User user) {
if (user != null) {
Log.d(TAG, "onChanged: " + user.getUsername());
}
}
});
}
That’s it.
Code for this article is here.
Video is here.
Twitter
Google+
Facebook
Reddit
LinkedIn
StumbleUpon
Email